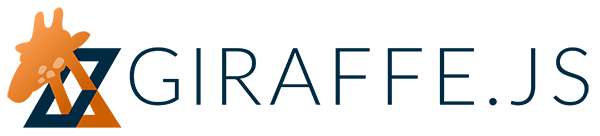
View Document Events
This example demonstrates Giraffe's document event bindings that link DOM events to Giraffe.View methods.
var View = Giraffe.View.extend({
template: '#view-template',
Giraffe.View provides a simple, convenient, and performant way to bind DOM
events to view method calls in your markup using the form
data-gf-eventName='viewMethodName'
. If the method isn't found on the view, it
searches up the hierarchy until it finds the method or fails on a view with no
parent
. In this template we bind to 'keyup'
and 'click'
events.
<script id="view-template" type="text/template">
<div id="hello-text">Hello world!</div>
<input type="text" value="world" data-gf-keyup="onChangeName">
<button data-gf-click="onAlertHello">Say hello in a popup</button>
</script>
By default, Giraffe binds only
click
and change
, and not keyup
as this
example uses, but you can easily set custom bindings using the class method
Giraffe.View.setDocumentEvents
.
Whenever the input changes via a 'keyup'
event, this method is called.
onChangeName: function(e) {
$('#hello-text').text('Hello ' + $(e.target).val() + '!');
},
Whenever the button is clicked, this method is called.
onAlertHello: function() {
alert($('#hello-text').text());
}
});
If you want to set your own custom DOM event bindings, this is how you'd do it.
Giraffe.View.setDocumentEvents(['mousedown', 'change', 'keyup']); // as an array
Giraffe.View.setDocumentEvents('mousedown change keyup'); // or as a single string
Giraffe.View.setDocumentEvents('click change'); // Giraffe's default events
Giraffe.View.setDocumentEvents('click keyup'); // the events for this example
Giraffe does not provide two-way data binding, but given its goal as a
light and flexible library, it should play nicely with most Backbone plugins.
This feature can be disabled by calling the class method
Giraffe.View.removeDocumentEvents()
.
All done! Let's create and attach the view.
var view = new View();
view.attachTo('body');
Try It
var View = Giraffe.View.extend({
template: '#view-template',
onChangeName: function(e) {
$('#hello-text').text('Hello ' + $(e.target).val() + '!');
},
onAlertHello: function() {
alert($('#hello-text').text());
}
});
Giraffe.View.setDocumentEvents(['mousedown', 'change', 'keyup']); // as an array
Giraffe.View.setDocumentEvents('mousedown change keyup'); // or as a single string
Giraffe.View.setDocumentEvents('click change'); // Giraffe's default events
Giraffe.View.setDocumentEvents('click keyup'); // the events for this example
var view = new View();
view.attachTo('body');
<!DOCTYPE html>
<html>
<head>
<link rel='stylesheet' type='text/css' href='../css/reset.css' />
</head>
<body>
<script id="view-template" type="text/template">
<div id="hello-text">Hello world!</div>
<input type="text" value="world" data-gf-keyup="onChangeName">
<button data-gf-click="onAlertHello">Say hello in a popup</button>
</script>
<script src="http://code.jquery.com/jquery-1.9.1.min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.4.4/underscore-min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/backbone.js/1.0.0/backbone-min.js"></script>
<script src="../backbone.giraffe.js" type="text/javascript"></script>
<script type='text/javascript' src='documentevents0-script.js'></script>
</body>
</html>